C Tutorial: An important role of fgets() and fputs()
fgets() and fputs():
Normally in our college when we are taught about the FILE MANAGEMENT topic in C, we come across these functions. We are told that fgets() is used to "get a string from a file" and fputs() is used to "put the string to a file".
This is not just the only use of these functions. There is another use and a very important use of these functions. Before coming to that, please have a look at this strings program
In this program, I've used gets() to take the input. Many of you might've noticed that, even though the index is just 10, we are able to input as many characters as possible whereas the program was supposed to take 9 characters + 1 string terminator (NULL character). This happens because, there is a lot of free space available in the buffer, hence, the program uses that space to take up the input, But, after some extent the buffer gets OVERLOADED and hence it doesn't produce the desired output. Instead, the program shows a error message and gets closed automatically. This is the major drawback of this program, as user doesn't know the size of character array being used and they may enter more characters than required.
example:
Input for the program...
Normally in our college when we are taught about the FILE MANAGEMENT topic in C, we come across these functions. We are told that fgets() is used to "get a string from a file" and fputs() is used to "put the string to a file".
This is not just the only use of these functions. There is another use and a very important use of these functions. Before coming to that, please have a look at this strings program
#include#include #include int main() { char input[10]; // character arrray with index 10 printf("Enter the input: \n"); gets(input); printf("\n\nInput is: \n"); puts(input); getch(); return 0; }
In this program, I've used gets() to take the input. Many of you might've noticed that, even though the index is just 10, we are able to input as many characters as possible whereas the program was supposed to take 9 characters + 1 string terminator (NULL character). This happens because, there is a lot of free space available in the buffer, hence, the program uses that space to take up the input, But, after some extent the buffer gets OVERLOADED and hence it doesn't produce the desired output. Instead, the program shows a error message and gets closed automatically. This is the major drawback of this program, as user doesn't know the size of character array being used and they may enter more characters than required.
example:
Input for the program...
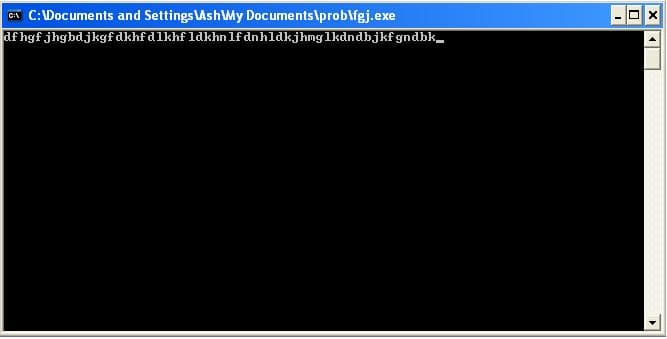
Error after execution....
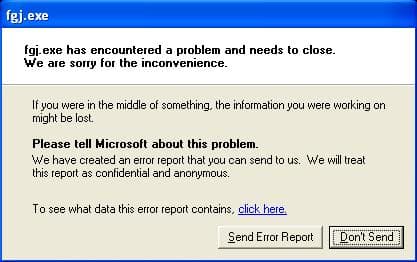
So, to overcome this problem, fgets() and fputs() play an important role. The only difference between the gets() and fgets() is the syntax. To get the input from the keyboard using fgets() the following syntax is followed.
fgets(input, sizeof(input), stdin);Similarly, for fputs(),
fputs(input, sizeof(input), stdout);so, the above code snippet can now be written as,
#includeNow, As we can see in the output, since, the size of input is 10, the output prints only 10 characters (9+1) without overloading the buffer and program executes without causing any trouble!!! 😀#include #include int main() { char input[10]; // character arrray with index 10 printf("Enter the input: \n"); fgets(input, sizeof(input), stdin); // stdin is buffer input printf("size of input is %d", sizeof(input)) printf("\n\nInput is: \n"); fputs(input, sizeof(input), stdout); // stdout is buffer output getch(); return 0; }
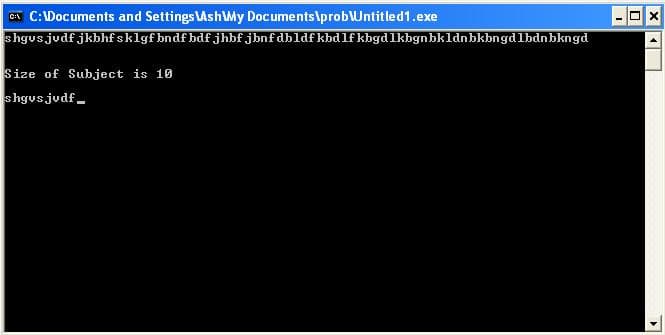
Replies
You are reading an archived discussion.
Related Posts
not familiar with car engines.........If the volumetric efficiency of an engine remains constant, and the carburetion is adjusted to maintain the same air-fuel ratio, how can we determine the percentage...
I wanna to know whether any website for free downloading of PLC software.
We used to have a dedicated section for books, but we decided to merge it with the Chit-Chat section because of inactivity. Now that we are bigger I see a...
During the patent infringement dispute between Samsung and Apple; Tim Cook, CEO of Apple & Larry Page, CEO of Google had a long secret chat on phone last week.
Larry...
I am saranya.. I am doing my fourth year M.Sc. Information Technology at Anna University.. When I was searching for some good placement preparation I saw this.. I am so...