C Programming: How to Call Another Program from Main Program?
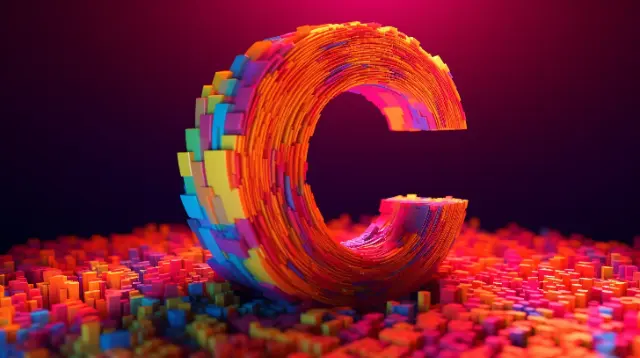
Hi CEans, in C language, is there any possibility to call another program from main program?
To call another program from a C program, you can use the system()
function which is available in the stdlib.h
library. The system()
function executes a system shell command. It creates a shell command process, executes the command, and then returns once the command has been completed.
Here is a simple example demonstrating how to use the system()
function to call another program:
#include /* Including standard library header files */
int main() {
int return_value;
/* The system() function takes a string argument that is the command to be executed.
In this case, the command is the path to the other program that you want to run. */
return_value = system("/path/to/other/program");
/* The system() function returns an integer. This value can be used to determine if the system call was successful.
Usually, if the value is -1, it means that the system call failed. */
if(return_value == -1) {
/* Handle the error accordingly. Here, we just simply print out a message. */
printf("System call failed");
}
return 0;
}
In the above example, replace /path/to/other/program
with the path to the executable that you wish to run.
Please be aware that this is a simple example. The system()
function has limitations and potential security risks because it invokes a shell command.
The shell can interpret special characters or environment variables, potentially leading to unexpected behavior if you pass in untrusted user input.
For more controlled execution of another program, look into fork()
and exec()
functions. These functions give you more control over input, output, and error streams, and avoid invoking a shell. However, they're also more complex to use.
Also, error handling for system()
calls should be more robust in a production environment.
Error messages should be descriptive and possibly logged for troubleshooting. The error handling in this example is kept simple for demonstration purposes.
Replies
-
saurabh2486Re: C program doubt..
i think yes its possible -
sherya mathurRe: C program doubt..
I will also go with saurabh
yes it is possible in c language to call another program from main program -
gaurav.bhorkarRe: C program doubt..
saurabh2486i think yes its possible
Can we know how this is done?sherya mathurI will also go with saurabh
yes it is possible in c language to call another program from main program -
ms_csRe: C program doubt..
Just make that file as header file and include it in the new file in which you want to use that file..thats it -
bharathkumarpRe: C program doubt..
will you please provide me simple program code.... -
gaurav.bhorkarRe: C program doubt..
Ah! now I remember,
If you want to run a program within a terminal using a C program, you can use the standard library function system ().
Suppose we want to run the program "hello.exe", here's how we can do this,
int main () { printf ("Before calling a program \n"); system ("hello.exe"); printf ("After calling the program \n"); getch (); return 0; }
-
bharathkumarpRe: C program doubt..
hi Gaurav, i executed the code which are provided by you.. It's executing only the printf statements but it not executing the block which are inside system.... Is there any header i need to include???? -
gaurav.bhorkarRe: C program doubt..
Yes, you have to include stdlib.h for system() to work.bharathkumarphi Gaurav, i executed the code which are provided by you.. It's executing only the printf statements but it not executing the block which are inside system.... Is there any header i need to include???? -
bharathkumarpRe: C program doubt..
HI Gaurav, this is also not working.. Only the printf statements are working...Without stdlib.h also it's showing the same output.... -
mayank1055yup it's not working
-
gaurav.bhorkarIt works perfectly in Visual C++ Express Edition 2008.
-
bharathkumarpok, i'll check it out... Thanks for the code...
-
silverscorpionDid you create the hello.exe file? Do you have it in the same directory?
-
gaurav.bhorkar
Yes the hello.exe file and our calling program's file should be in the same directory.silverscorpionDid you create the hello.exe file? Do you have it in the same directory?
The hello.exe must be created, it can have anything like printf ("Hello World \n"). -
bharathkumarpya i put the file inside the same directory,but it is not working.....
-
gaurav.bhorkar
Which compiler are you using?bharathkumarpya i put the file inside the same directory,but it is not working.....
If you are using VC++, then your program's executable is in the debug directory. That is, My Documents/Visual Studio/Projects//Debug.
Now paste the hello.exe into this directory.
You are reading an archived discussion.