TUTORIAL: ERROR HANDLING IN C
Beginners Tutorial: Handling errors in C ( Use of errno.h )
Hello CEans! In the following tutorial, you will learn how to handle the wrong data entered by users.
INTRODUCTION:
There are many exceptions regarding the range of a particular datatype in C. Each datatype has a certain range, and if the range is exceeded, the compiler fails to recognize the datatype and produces a garbage value to the variable (to know about garbage values in C, #-Link-Snipped-#) and hence, departs from the expected output and sometimes the program even crashes. Definitely, we don't want our software to crash (taking in consideration of big projects). So, to handle these errors or wrong data or to display alerts when the wrong data is entered, we use error exception concept.
EXAMPLE:
Let us consider the following code snippet of fibonacci series. Normally, people write the fibonacci
sequence program, as shown below.
The program produces perfect output for many ranges. But there are some exceptions.
1) What if the range is -ve?
2) What if the range is less than or equal to 1?
3) What if the range is big?? Suppose above 50?
These are the outputs produced for the above exceptions, which are surely not the outputs
which we expected.
HOW TO OVERCOME:
These are the steps to be followed to overcome these exceptions!
1) checking the condition for range.
2) printing the error.
3) Re- starting the program to get a desired range.
1> To check the error.
we all know we can use conditional statements if-else. To know about it <a href="https://www.tutorialspoint.com/ansi_c/c_control_statements.htm" target="_blank" rel="nofollow noopener noreferrer">C - Flow Control Statements</a>
2> Printing the error.
So, this is what the tutorial is all about. How to print the error??
Using printf() ?? No, Why to type our own error statements, when the C environment provides the inbuilt error statements.
Just include the <errno.h> header file and print the error using perror(). 😀
To use perror(), you must know what error statements will be printed for whant kind of error. Have a look at the <errno.h> in my system. This may vary in your system.
For, the program of fibonacci sequence the error code I will be using is EINVAL and ERANGE
For using this, you just need to declare a few things as,
You are even allowed to write a small statement in perror() as
3> Re-starting the program to get a desired range.
This can be done in two ways! Using goto or by just using loops!
As goto causes ambiguity in big programs, we avoid using it.
IMPLEMENTATION IN PROGRAM:
So after knowing about handling the exceptions, we just implement it in our program as below.. 😀
OUTPUT FOR THE THREE EXCEPTIONS:
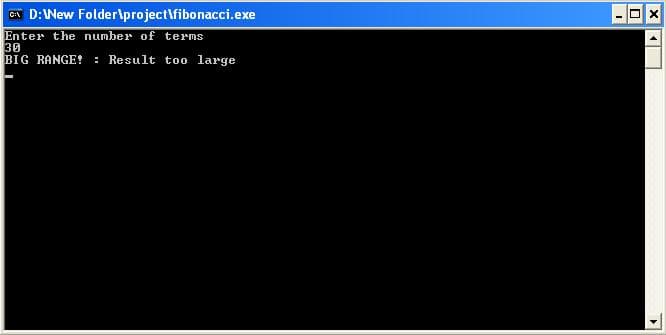
Hello CEans! In the following tutorial, you will learn how to handle the wrong data entered by users.
INTRODUCTION:
There are many exceptions regarding the range of a particular datatype in C. Each datatype has a certain range, and if the range is exceeded, the compiler fails to recognize the datatype and produces a garbage value to the variable (to know about garbage values in C, #-Link-Snipped-#) and hence, departs from the expected output and sometimes the program even crashes. Definitely, we don't want our software to crash (taking in consideration of big projects). So, to handle these errors or wrong data or to display alerts when the wrong data is entered, we use error exception concept.
EXAMPLE:
Let us consider the following code snippet of fibonacci series. Normally, people write the fibonacci
sequence program, as shown below.
#include <stdio.h> int main() { int range, i, a = 0, b = 1, c; printf("Enter the range: "); scanf("%d",&range); printf("Fibonacci sequence for %d range is \n\n",range); printf("%d %d ",a,b); for(i = 3; i <= range; i++) { c = a+ b; a = b; b = c; printf("%d ",c); } fflush(stdin); getchar(); return 0; }
The program produces perfect output for many ranges. But there are some exceptions.
1) What if the range is -ve?
2) What if the range is less than or equal to 1?
3) What if the range is big?? Suppose above 50?
These are the outputs produced for the above exceptions, which are surely not the outputs
which we expected.
HOW TO OVERCOME:
These are the steps to be followed to overcome these exceptions!
1) checking the condition for range.
2) printing the error.
3) Re- starting the program to get a desired range.
1> To check the error.
we all know we can use conditional statements if-else. To know about it <a href="https://www.tutorialspoint.com/ansi_c/c_control_statements.htm" target="_blank" rel="nofollow noopener noreferrer">C - Flow Control Statements</a>
2> Printing the error.
So, this is what the tutorial is all about. How to print the error??
Using printf() ?? No, Why to type our own error statements, when the C environment provides the inbuilt error statements.
Just include the <errno.h> header file and print the error using perror(). 😀
To use perror(), you must know what error statements will be printed for whant kind of error. Have a look at the <errno.h> in my system. This may vary in your system.
#define EPERM 1 /* Operation not permitted */ #define ENOFILE 2 /* No such file or directory */ #define ENOENT 2 #define ESRCH 3 /* No such process */ #define EINTR 4 /* Interrupted function call */ #define EIO 5 /* Input/output error */ #define ENXIO 6 /* No such device or address */ #define E2BIG 7 /* Arg list too long */ #define ENOEXEC 8 /* Exec format error */ #define EBADF 9 /* Bad file descriptor */ #define ECHILD 10 /* No child processes */ #define EAGAIN 11 /* Resource temporarily unavailable */ #define ENOMEM 12 /* Not enough space */ #define EACCES 13 /* Permission denied */ #define EFAULT 14 /* Bad address */ /* 15 - Unknown Error */ #define EBUSY 16 /* strerror reports "Resource device" */ #define EEXIST 17 /* File exists */ #define EXDEV 18 /* Improper link (cross-device link?) */ #define ENODEV 19 /* No such device */ #define ENOTDIR 20 /* Not a directory */ #define EISDIR 21 /* Is a directory */ #define EINVAL 22 /* Invalid argument */ #define ENFILE 23 /* Too many open files in system */ #define EMFILE 24 /* Too many open files */ #define ENOTTY 25 /* Inappropriate I/O control operation */ /* 26 - Unknown Error */ #define EFBIG 27 /* File too large */ #define ENOSPC 28 /* No space left on device */ #define ESPIPE 29 /* Invalid seek (seek on a pipe?) */ #define EROFS 30 /* Read-only file system */ #define EMLINK 31 /* Too many links */ #define EPIPE 32 /* Broken pipe */ #define EDOM 33 /* Domain error (math functions) */ #define ERANGE 34 /* Result too large (possibly too small) */ /* 35 - Unknown Error */ #define EDEADLOCK 36 /* Resource deadlock avoided (non-Cyg) */ #define EDEADLK 36 /* 37 - Unknown Error */ #define ENAMETOOLONG 38 /* Filename too long (91 in Cyg?) */ #define ENOLCK 39 /* No locks available (46 in Cyg?) */ #define ENOSYS 40 /* Function not implemented (88 in Cyg?) */ #define ENOTEMPTY 41 /* Directory not empty (90 in Cyg?) */ #define EILSEQ 42 /* Illegal byte sequence */These are the error codes provided for various problems in the programming.
For, the program of fibonacci sequence the error code I will be using is EINVAL and ERANGE
For using this, you just need to declare a few things as,
errno = EINVAL; // errno is inbuilt name. No need to be declared in main() perror(NULL); // prints the error for EINVALSimilarly,
errno = ERANGE; perror(NULL);
You are even allowed to write a small statement in perror() as
perror("BIG RANGE");The output for this error will print "BIG RANGE: result too large"
3> Re-starting the program to get a desired range.
This can be done in two ways! Using goto or by just using loops!
As goto causes ambiguity in big programs, we avoid using it.
IMPLEMENTATION IN PROGRAM:
So after knowing about handling the exceptions, we just implement it in our program as below.. 😀
#include <errno.h> #include <stdio.h> #include <stdlib.h> int main() { int n, a = 0, b = 1, c, i, done = 0; while(!done) /* error detection loop. If detected, program restarts*/ { printf("Enter the number of terms\n"); scanf("%d",&n); if(n <= 0) { errno = EINVAL; perror("Range must be positive"); fflush(stdin); getchar(); done = 0; system("cls"); } else if(n > 20) { errno = ERANGE; perror("BIG RANGE! "); fflush(stdin); getchar(); done = 0; system("cls"); } else done = 1; } printf("First %d terms of Fibonacci series are : \n",n); if(n>1) { printf("%d %d ",a,b); for ( i = 2 ; i < n ; i++ ) { c = a + b; a = b; b = c; printf("%d ",c); } } else printf("0"); fflush(stdin); getchar(); return 0; }
OUTPUT FOR THE THREE EXCEPTIONS:
0